susan
You need 3 min read
Post on Feb 09, 2025
Table of Contents
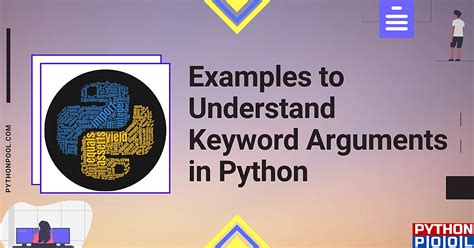
Mastering Keyword Arguments in Python: A Comprehensive Guide
Python's flexibility shines through its support for keyword arguments, a powerful feature that enhances code readability, maintainability, and robustness. Understanding keyword arguments is crucial for writing clean, efficient, and Pythonic code. This comprehensive guide will delve into the intricacies of keyword arguments, illustrating their usage with practical examples and best practices.
What are Keyword Arguments?
Keyword arguments, also known as named arguments, allow you to pass arguments to a function by specifying the parameter name explicitly. This contrasts with positional arguments, where the order of arguments matters. Keyword arguments provide several advantages:
- Improved Readability: Code becomes more self-documenting as the purpose of each argument is clearly stated.
- Flexibility in Argument Order: You can pass arguments in any order as long as you specify the parameter names.
- Default Values: You can define default values for parameters, making functions more versatile.
- Optional Arguments: Keyword arguments simplify handling optional inputs.
Syntax and Usage
The syntax for using keyword arguments is straightforward. When calling a function, you specify the parameter name followed by an equals sign (=
) and the value.
def greet(name, greeting="Hello"):
print(f"{greeting}, {name}!")
greet(name="Alice", greeting="Good morning") # Output: Good morning, Alice!
greet(greeting="Hi", name="Bob") # Output: Hi, Bob! Order doesn't matter!
greet(name="Charlie") # Output: Hello, Charlie! Uses default greeting
In this example, name
is a required positional argument, while greeting
is a keyword argument with a default value of "Hello".
Keyword Arguments vs. Positional Arguments
The key difference lies in how arguments are passed:
- Positional Arguments: The order of arguments is strictly enforced. The first argument is assigned to the first parameter, the second to the second, and so on.
- Keyword Arguments: The order is irrelevant; the argument's name explicitly specifies which parameter it's assigned to.
Combining Positional and Keyword Arguments
Python allows you to combine both positional and keyword arguments in a function call. However, positional arguments must come before keyword arguments.
def describe_pet(animal_type, pet_name, age=None):
print(f"\nI have a {animal_type}.")
print(f"My {animal_type}'s name is {pet_name.title()}.")
if age:
print(f"My {animal_type} is {age} years old.")
describe_pet('hamster', 'harry', age=2) # Correct usage
describe_pet('dog', 'willie', 6) # Correct usage - age is positional
#describe_pet(pet_name='harry', 'hamster') # Incorrect - Positional arg after keyword arg
Keyword-Only Arguments
In Python 3, you can define parameters that must be passed as keyword arguments. This improves code clarity and prevents accidental misinterpretations. You achieve this by placing a single *
in the parameter list before the keyword-only arguments.
def calculate_area(length, width, *, units="meters"):
area = length * width
print(f"The area is {area} {units}")
calculate_area(5, 10) #units defaults to "meters"
calculate_area(5, 10, units="feet") # Correct use of keyword-only arg
#calculate_area(5, 10, "feet") # Incorrect - units must be keyword arg
units
is now a keyword-only argument.
Practical Applications
Keyword arguments are invaluable in various scenarios:
- Complex Functions: Enhance readability when dealing with numerous parameters.
- APIs and Libraries: Provide a clear and consistent interface for users.
- Configuration Settings: Allow flexible customization through named parameters.
Best Practices
- Use descriptive parameter names to enhance code clarity.
- Provide default values for optional arguments.
- Leverage keyword-only arguments to enforce proper usage.
- Document your function parameters clearly, specifying whether they are positional or keyword arguments.
By mastering keyword arguments, you'll write more elegant, readable, and maintainable Python code. They are an essential tool in any Python developer's arsenal.
Thanks for visiting this site! We hope you enjoyed this article.